Basic raspberry pi fan control with n channel/ p channel mosfet
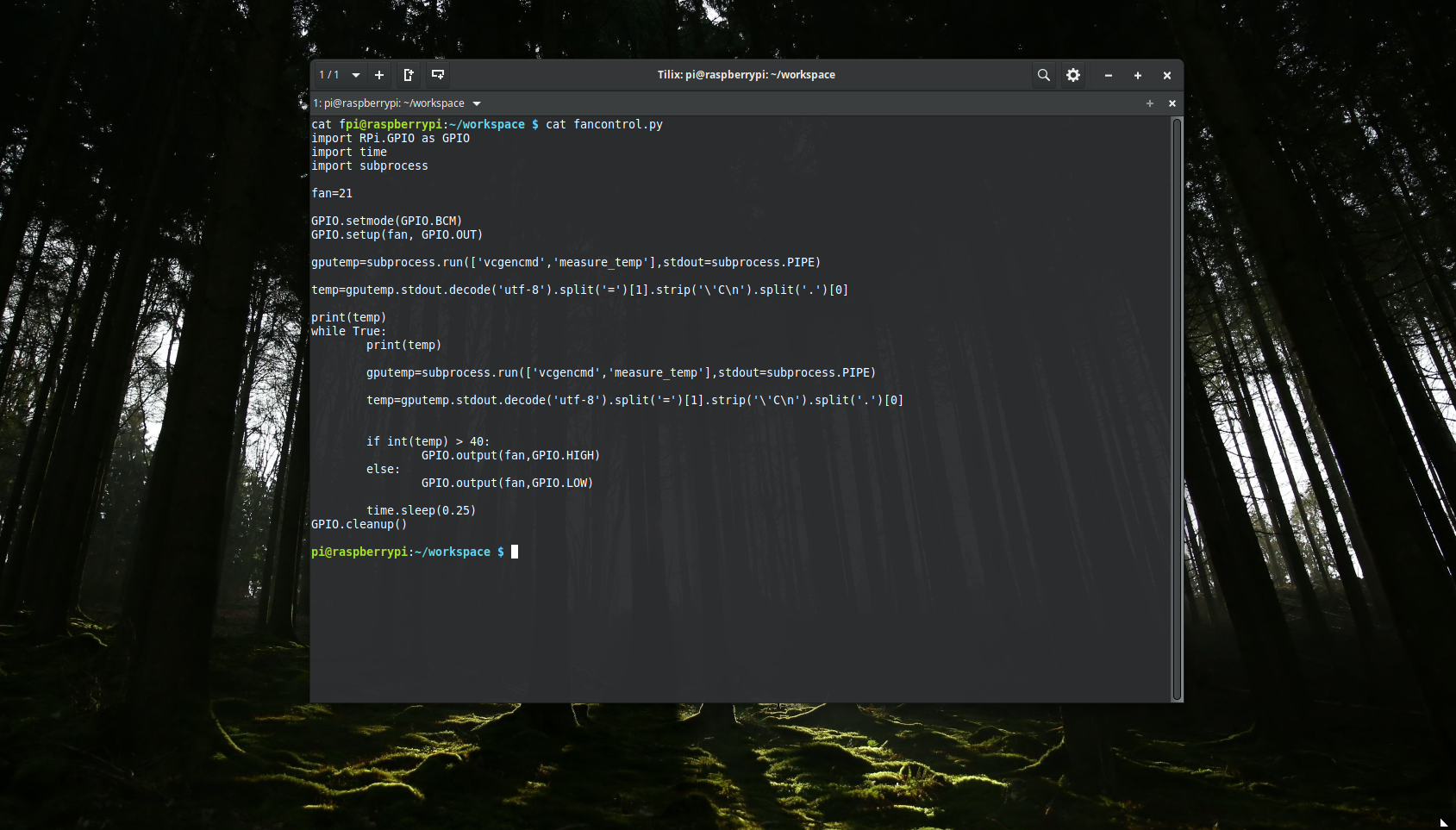
to add a fan to your raspberry pi you can wire it directly to your 5v and ground, but to control it based on temperature you need to use a mosfet or a transistor, your gpio pins are not powerful enough to switch on a fan
Mosfets
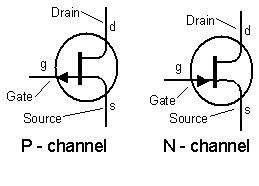
there are 2 main types of mosfets p channel and n channel
with an p channel mosfet the source is connected to ground, the drain to the load, and it will turn on when a positive voltage is applied to the gate
with an n channel mosfet the source is connected to a positive voltage, and it will turn on when the voltage on the gate is below the source voltage
in this example we use a n channel mosfet but you can do the same with your p chanel mosfet see the hint downbelow
Connections
connect the mosfet gate to the raspberry pi gpio pin 21
connect the gound lead from your fan to drain
and connect the mosfet source to ground
Software
import RPi.GPIO as GPIO
import time
import subprocess
fan=21
GPIO.setmode(GPIO.BCM)
GPIO.setup(fan, GPIO.OUT)
gputemp=subprocess.run(['vcgencmd','measure_temp'],stdout=subprocess.PIPE)
temp=gputemp.stdout.decode('utf-8').split('=')[1].strip('\'C\n').split('.')[0]
print(temp)
while True:
print(temp)
gputemp=subprocess.run(['vcgencmd','measure_temp'],stdout=subprocess.PIPE)
temp=gputemp.stdout.decode('utf-8').split('=')[1].strip('\'C\n').split('.')[0]
if int(temp) > 40:
GPIO.output(fan,GPIO.HIGH)
else:
GPIO.output(fan,GPIO.LOW)
time.sleep(0.25)
GPIO.cleanup()